Conditional Flow Control Structures in R and Python
Comparison between R and Python syntax for executing commands like if, ifelse, and elif.
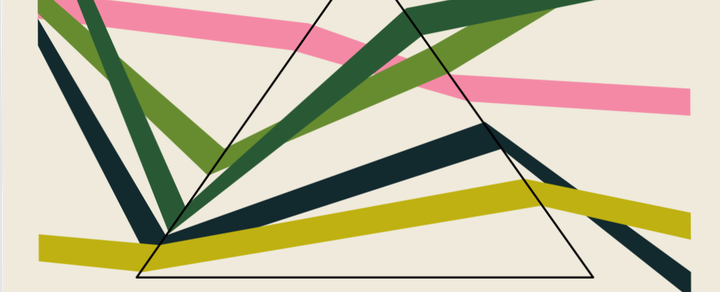
Conditional flow control structures are used to execute certain code when certain conditions are met (or not), which will be evaluated as true or false. There are three possible conditions: - If A occurs, then B occurs. - If A occurs, then B occurs; else occurs C. - If A occurs, then B occurs; else, if C occurs then D occurs; else occurs E.
In both languages, the keywords for those three situations are if
, if...else
and elif/else if
respectively. Beyond that, the biggest difference between the two is the syntax. Let’s look at each case.
if conditional
Suppose we ask the user to enter their age to test if they are of legal age. In R, the conditional looks like this:
age <- as.numeric(readline(prompt = "Write your age: "))
if (age >= 18){
print("User is of legal age.")
}
In this case, the structure only evaluates if the user is of legal age, in which case it warns him with the proposed phrase. But since it does not specify what to do if the statement is evaluated as FALSE
, the code does not return anything when the user is not of legal age.
In Python, this same block looks like this:
age = int(input("Write your age: "))
if age >= 18:
print("User is of legal age.")
As we can see, both blocks of code are quite similar. The big difference is that in R the statements to be evaluated must be enclosed in parentheses, and the code that is executed must be enclosed in braces.
if…else conditional
If we need to specify a different code for when the evaluated statement is FALSE
, we use the else
function. In R:
if (age >= 18){
print("User is of legal age.")
} else {
print("User is not of legal age.")
}
R also has the ifelse()
function that combines that sequence into a single line of code, where the first argument is the statement to evaluate, the second is the result in the TRUE
case, and the third is the result in the FALSE
case:
ifelse(age >= 18, "User is of legal age.", "User is not of legal age.")
In Python:
if age >= 18:
print("User is of legal age.")
else:
print("User is not of legal age.")
elif Conditional
In some cases, in addition to establishing the code to be executed in case the statement is true or false, we need to create a chain of conditions. For that, R combines the two previous tools.
if (age >= 18){
print("User is of legal age.")
} else if (age >= 16){
print("User is not of legal age, but they can vote in Argentina.")
} else {
print("User is not of legal age nor can they vote in Argentina.")
}
In Python, this is done with the elif
expression, which is supplemented by else
to indicate the case when both conditions are false.
if age >= 18:
print("User is of legal age.")
elif age >= 16:
print("User is not of legal age, but they can vote in Argentina.")
else:
print("User is not of legal age nor can they vote in Argentina.")
Closing
In summary, we have explored the conditional flow control structures in R and Python. Through examples and comparisons, we have seen how each language handles conditions and conditional statements. We hope this post has provided you with a solid understanding of how to use these structures in your programming projects.
As always, remember you can suscribe to my blog to stay updated, and if you have any questions, don’t hesitate to contact me. And if you like what I do, you can buy me a cafecito from Argentina or a kofi.