Iterative control flow structures in R and Python
In this post we are going to compare how iterative control flow structures are implemented in R and Python.
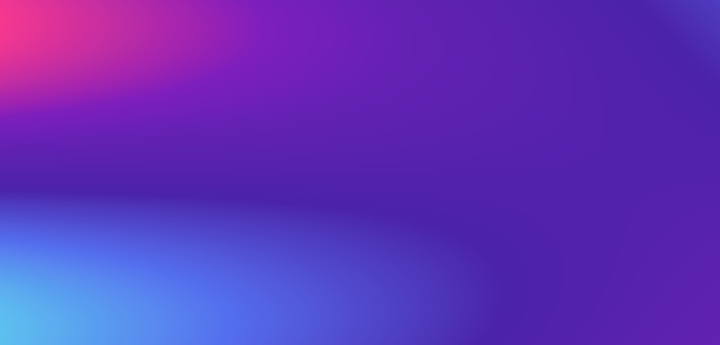
Iterative control structures or loops allow you to execute a block of code repeatedly as long as a certain condition is satisfied. There are two major loops: for and while. Let’s see the difference in the syntax of R and Python.
For loop
The for loop is used to iterate a code in a data set, which can be a list or an array-like object. For example, if we want to print a list of numbers from 1 to 10, we can do it like this in R:
for (i in 1:10){
print(paste("The number is", i))
}
## [1] "The number is 1"
## [1] "The number is 2"
## [1] "The number is 3"
## [1] "The number is 4"
## [1] "The number is 5"
## [1] "The number is 6"
## [1] "The number is 7"
## [1] "The number is 8"
## [1] "The number is 9"
## [1] "The number is 10"
As we saw in the previous post on conditional structures, in R the statements to evaluate or conditions are enclosed in parentheses, while the code to be executed is enclosed in braces. In contrast, in Python the syntax is defined exclusively by the indentation and the colon:
for i in range(1, 10):
print(f"The number is {i}")
## The number is 1
## The number is 2
## The number is 3
## The number is 4
## The number is 5
## The number is 6
## The number is 7
## The number is 8
## The number is 9
In addition to the syntax, it’s important to note that R uses 1-based indexing, while Python uses 0-based indexing. This means that if you want to iterate from 1 to 10 in Python, you need to specify the range as range(1, 11)
, otherwise it will iterate from 0 to 9.
Loops can iterate through elements within a list, like so:
vowels <- c("a", "e", "i", "o", "u")
for (i in vowels){
print(paste("The letter", i, "is a vowel."))
}
## [1] "The letter a is a vowel."
## [1] "The letter e is a vowel."
## [1] "The letter i is a vowel."
## [1] "The letter o is a vowel."
## [1] "The letter u is a vowel."
Unlike R, in Python strings work like arrays, so to run this code above we don’t need to create the vowels
element first:
for i in "aeiou":
print(f"The letter {i} is a vowel.")
## The letter a is a vowel.
## The letter e is a vowel.
## The letter i is a vowel.
## The letter o is a vowel.
## The letter u is a vowel.
While loop
The while loop is used to indicate that a block of code must be executed while a certain condition is met.
i <- 2020
while (i >= 2020 & i <= 2022) {
print(paste("The year", i, "was pandemic"))
i <- i + 1
}
## [1] "The year 2020 was pandemic"
## [1] "The year 2021 was pandemic"
## [1] "The year 2022 was pandemic"
The loop starts with the i
pointer with the value 2020
; with each iteration, it increases by one unit. In cases where the value is greater than or equal to 2020 and less than or equal to 2022, the requested phrase will be printed.
In Python, the sequence is similar, but without the braces and parentheses, and replacing the ampersand (&) with the keyword and
:
i = 2020
while i >= 2020 and i <= 2022:
print(f"The year {i} was pandemic.")
i += 1
## The year 2020 was pandemic.
## The year 2021 was pandemic.
## The year 2022 was pandemic.
Break and continue loops
In some situations we need to include some condition that allows to break a loop, which is used with the break statement:
i <- 1
while (i < 20){
if (i == 18){
break
}
print(i)
i <- i + 1
}
## [1] 1
## [1] 2
## [1] 3
## [1] 4
## [1] 5
## [1] 6
## [1] 7
## [1] 8
## [1] 9
## [1] 10
## [1] 11
## [1] 12
## [1] 13
## [1] 14
## [1] 15
## [1] 16
## [1] 17
In Python, the sequence is similar, with the aforementioned differences in syntax around the use of parentheses and braces:
i = 1
while i < 20:
if i == 18:
break
print(i)
i += 1
## 1
## 2
## 3
## 4
## 5
## 6
## 7
## 8
## 9
## 10
## 11
## 12
## 13
## 14
## 15
## 16
## 17
However, sometimes we just need to skip the iteration of a particular condition. This is executed with the statement next in R and continue in Python:
i <- 0
while (i < 10){
i <- i + 1
if (i == 5){
next
}
print(i)
}
## [1] 1
## [1] 2
## [1] 3
## [1] 4
## [1] 6
## [1] 7
## [1] 8
## [1] 9
## [1] 10
The result of this code is the sequence 1-2-3-4-6-7-8-9-10, that is, the presentation of the data on the screen skips the 5. On the other hand, in Python, the same thing is generated this way:
i = 1
while i < 10:
i += 1
if i == 5:
continue
print(i)
## 2
## 3
## 4
## 6
## 7
## 8
## 9
## 10
Conclusions
Even though as we learn more complex syntax the differences between R and Python grow, I think this is a very useful approach to learn a new language. Building over our previous knowledge allows us to relate the new information to something we already have established.
I hope this was useful to you. As always, remember you can suscribe to my blog to stay updated, and if you have any questions, don’t hesitate to contact me. And if you like what I do, you can buy me a cafecito from Argentina or a kofi.